Libraries and frameworks for a budding front end developer
by Cameron Tebbenham-Small, Design Enterprise Studio Member, March 2022
So, you’ve made your first website, maybe even a few, but now you want to look ahead and advance to an intermediate level. Let me introduce you to the wonderful world of CSS and JavaScript libraries frameworks by exploring a few that I have used in my own workflows. But firstly, what are frameworks and libraries and how do they differ?
A coding library is a collection of standard functionalities – pieces of reusable code that enable developers to skip a great many steps that have likely already been accomplished by developers before them. These could be maths functions, interactive web-forms or other that are then inserted into your code as a supplementary function. Think of it like adding an extra chair to your room – you’ve already done some decorating, but don’t want to build a full new Ikea chair so get something premade that fits the job.
If a library is like a piece of furniture, a framework is the entire room – if not the entire house. A framework sets the shape and layout of your website/webapp in a fundamental way. They are the scaffolding for your entire project, which more often than not may happen to also contain libraries inside of it. This is extremely useful if you intend to make things like single page applications with optimised UI rendering, want to neatly structure every aspect of your project or don’t want to code every single function from scratch.
As a front-end developer, I’ll be focusing on a couple different frameworks for both CSS and JavaScript – but will for the most part skip explanations about back-end support.
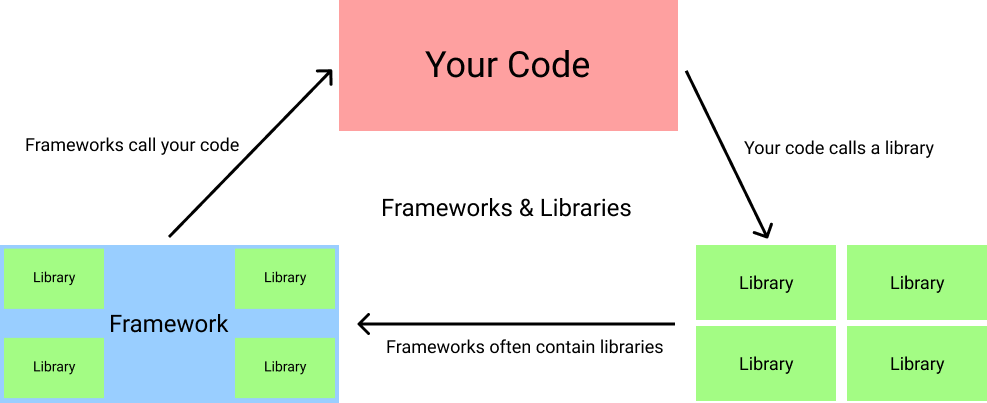
CSS
CSS frameworks can be boiled down into two main categories – those that pre-style elements and those that don’t. The former is often easier to learn, while the latter offers more choice in how you style your elements. As such I will discuss one of each that I have personally used.
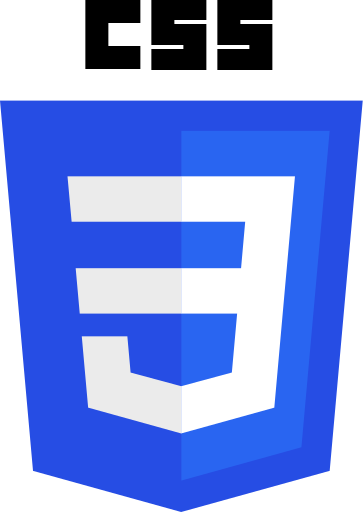
Bootstrap
You cannot search for a CSS framework without seeing Bootstrap, and for good reason. Initially branded “Twitter Blueprint” and created by a couple of developers at Twitter to encourage styling consistency, it became often source and freely available in 2011. That is, essentially, the purpose of all CSS frameworks – consistency. The more consistent your styling within a website, the better the flow of navigation, information and efficiency for the end user.
Benefits
Bootstrap’s own website describes itself as being a customisable “responsive mobile-first” tool that features grid systems, prebuilt components and JavaScript plugins. It even features support for Sass – an extension for CSS that enables a host of extra functionality such as in-CSS nesting, mixins etc, a potential topic for further reading. Bootstrap is highly desirable for its ease of use and responsive structure, as evident in the fact that according to Stack Share even in 2022, over 50,000 companies use bootstrap. These include big names such as Twitter, Udemy, Spotify, Snapchat and more.
“Bootstrap is highly desirable for its ease of use and responsive structure”
Bootstrap is also one of the easiest CSS frameworks to dive into as as many of the elements (such as buttons) come pre-styled. Documentation is extensive and Bootstrap has a vibrant community that is always coming up with new themes and styles.
Drawbacks
However, with that ease of entry comes a few limitations. Firstly, there is a saying that once you’ve seen one Bootstrap site – you’ve seen them all. While not necessarily true, it is harder to make each site look and feel distinct. This is because of all that pre-styling previously discussed. Further, unless you use the minified version, you will end up with a much larger CSS file containing hundreds of classes that you will never use. While you can of course set up your own external CSS file and override some of Bootstrap’s presets, with or without the dreaded “!important” tag (such as changing danger/primary/secondary for main colours), developers who prefer granularity in their design might want to stay away.
This leads me to the second CSS framework and perhaps my new favourite…
Tailwind
Tailwind is all about efficiently writing vanilla CSS inline with your HTML in a way that gives freedom to the developer. In fact, Tailwind’s motto is that “Most CSS frameworks do too much”. This results in a lightweight framework that only gives you as much as you need to get the task done. CSS coding becomes mostly unnecessary, but you still need to know how CSS works. Tailwind is a framework that will grow with your understanding of CSS in way that is very rewarding for the active developer
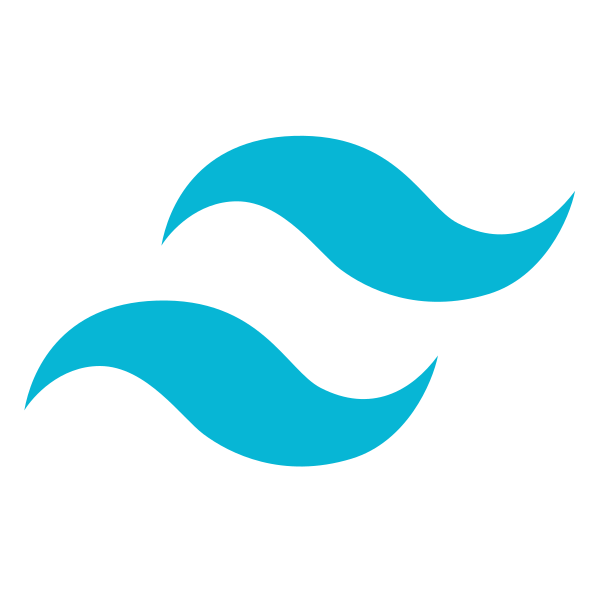
Tailwind’s motto is “most CSS frameworks do too much.”
Benefits
Tailwind differs from Bootstrap in that it doesn’t pre-style your content for you. Instead, it packages vanilla CSS into single line phrases and allows you to keep all your CSS inline without cluttering up your HTML files. What’s more, media breakpoints are easily incorporated by adding a prefix to whatever other class you want to use. For example, you could set the width to be 24 rem on a mobile device (w-24) and 48 on a medium sized screen (md:w-24). This also allows you to choose whether you want your project to be mobile or desktop first – unlike Bootstrap which is explicitly mobile-first.
This is a form of Atomic CSS, whereby an element’s classes denote how the element will look. If the element has a class list of “text-center text-white bg-black p-4” you can expect to see centred white text on a black background, with a padding of 4 rem. I particularly love the use of rem for sizing because it is tied to the root font size. This ensures that all your elements are scaled proportionate to each other. All these Tailwind Classes can be found in a handy, searchable cheat sheet: https://tailwindcomponents.com/cheatsheet/
Drawbacks
As previously mentioned, strong CSS knowledge is required to make the most use out of Tailwind, unless you make heavy use out of community pre-styled assets. Further, in my experience, Tailwind is harder to initially set up than Bootstrap for a few reasons.
Firstly, you’ll want to use PostCSS or Sass to make the most use out of all Tailwind’s features. In Visual Studio Code, this can be done by installing the PostCSS Language Support extension and installing postcss-cli via npm. You will also likely want to install Tailwind CSS IntelliSense at the same time as it autocompletes Tailwind classes similar to how Emmet functions.
Next, you’ll need to follow the Tailwind installation instructions and set up a config file which tells Tailwind which files to scan for its classes, and finally run the build/watch command to auto-generate the necessary CSS. It may sound complicated, but once you’ve done it the first time, you’ll be set.
JavaScript
React JS
Though it is often described as such, technically React JS is not a framework – it’s a library, albeit a very powerful one originally created by a Facebook engineer in 2011. Most articles tend to blur the line between frameworks and libraries – especially when React is involved – and it provides an excellent first step into what JavaScript frameworks look like.
At its core, front end development is all about portraying information and allowing for easy navigation between said information. React JS does this better than most and is highly in demand. As of 2021 React is the most used web framework/library among developers and is also the most desired by developers according to Statista and the 2021 Stackoverflow Developer Survey.
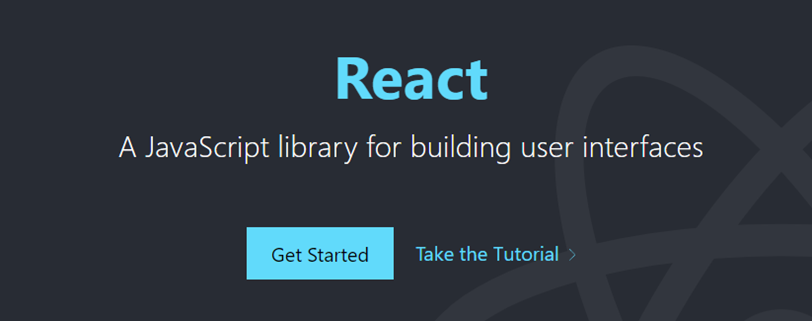
The Virtual DOM
It is specialized in the creation of sophisticated user interfaces and just as its name suggests, React reacts to changes in the Virtual DOM (Document Object Model) in a way that boosts performance and load times.
Normally, you would use HTML to update the DOM, but when you need to dynamically alter content on the page without constantly reloading the page, you need something more. React uses a form of JavaScript called JSX to selectively update the exact components that need updating – without needing to alter the entire DOM. This optimises the user interaction process from a technical standpoint, reducing load times and boosting performance.
Components
React JS uses reusable and declarative components to break down complex UIs into their base components. These can then be freely reused across the entire application. Similarly to how Tailwind and Bootstrap essentially let you write CSS inside your HTML files, JSX allows you to write full HTML inside your JavaScript files. This, when properly managed, can result in incredibly cleanly organised file structures where different elements of your web-app become self-contained and easily manageable components. No more scrolling through thousands of lines of code to find that one thing you need to change.
Community and Plugins
Lastly, React is constantly being updated and has a plethora of plugins and extra resources such as React Router, which enables powerful page routing despite the single page nature of React applications. This means that you can have a single index.html file that is dynamically updated with whatever content you need to show according to your JS files. If you liked the sound of Bootstrap for CSS, there is also a React specific variant called Reactstrap to sink your teeth into. More of a mobile fan? React Native has you covered, allowing you to convert your web-centric code into a fully usable mobile application.
Conclusion
That concludes my brief introduction to a handful of the most notable frameworks being used in the industry today. There are new solutions coming out all the time, which also pushes the leading frameworks to innovate and continue developing their tools. CSS frameworks can drastically speed up the development process when custom code isn’t required on a large scale, while a library such as React opens up doors to all sorts of new ways to develop for the web. Enjoy!
Bootstrap references:
https://getbootstrap.com/docs/5.1/components/button-group/
https://getbootstrap.com/docs/5.1/getting-started/introduction/
https://realtoughcandy.com/is-bootstrap-dead/
https://stackshare.io/bootstrap
Tailwind references:
https://tailwindcss.com/docs/installation
React references:
https://www.statista.com/statistics/1124699/worldwide-developer-survey-most-used-frameworks-web/
https://insights.stackoverflow.com/survey/2021#most-loved-dreaded-and-wanted-webframe-want
General References
https://www.freecodecamp.org/news/2019-web-developer-roadmap/
https://skillcrush.com/blog/javascript-frameworks-vs-libraries/